QR Code Generator in Android App using Zxing Library
- Susantini Behera
- Aug 13, 2020
- 3 min read
Recently I has been working on Visitors Management App where I realised the visitors information can be squizzed into QR code and when it is essential can be retrived back by the management authority inside any working Organization/Campus. Hence QR Code generation and reader process can be extremely helpful.
What is QR Code?
A QR code (curtailed from Quick Response code) is a sort of framework standardized tag (or two-dimensional scanner tag) first structured in 1994 for the car business in Japan. A standardized tag is a machine-coherent optical name that contains data about the thing to which it is joined.
Why QR Codes are more relevant in 2020?
A tremendous lump of organizations and brands of all sizes are moving towards an innovation empowered market, QR Codes are more significant than any other time in recent memory. The quantity of outputs has been expanding imperceptibly, along these lines going everybody to QR Codes.
How does it works?
Essentially, a QR code works similarly as a standardized tag at the grocery store.... Each QR code comprises of various dark squares and dabs which speak to specific snippets of data. At the point when your Smartphone checks this code, it make an interpretation of that data into something that can be effectively comprehend by people.
Generating a QR Code
To scan a QR Code the first and foremost required is itself a QR Code. So for this tutorial we will generate a Simple QR Code consist of Visitors Name and purpose of visit. Below is the QR Code I already generated a free service using simple steps as follows:

Figure 1:QR code
Steps to Generate QR Code:
create a new project in Android Studio named QRCode.
Adding Zxing Library for generating QR Code.So once your project is loaded go to your app level build.gradle file and add the following dependency.
implementation 'com.google.zxing:core:3.3.0'
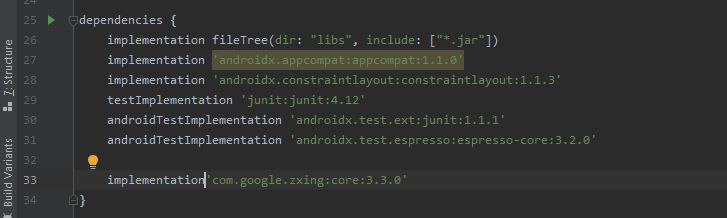
Then sync with the gradle.
creating user Interface containing the information of the visitor including the name and purpose of visit .It willl generate QR Code for the respective information.
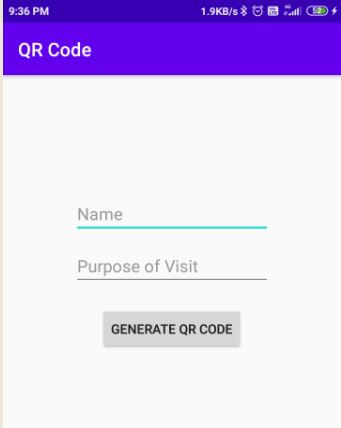
Figure 2:Android QR Code Generator
For designing the above layout you can use the following xml code.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="10dp"
android:gravity="center"
tools:context=".MainActivity">
<EditText
android:id="@+id/editTextTextPersonName3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="30dp"
android:ems="10"
android:inputType="textPersonName"
android:hint="Name"
/>
<EditText
android:id="@+id/editTextTextPersonName2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_gravity="center"
android:ems="10"
android:inputType="textLongMessage"
android:hint="Purpose of Visit"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button2" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:layout_marginTop="20dp"
android:onClick="QRCodeButton"
android:text="Generate QR Code"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:id="@+id/imageView"
android:layout_marginTop="10dp"
/>
</LinearLayout>
Now comes to java file for generating QR Code
It includes certain steps:
defining view objects
package coeaibbsr.in.qrcode;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Bitmap;
import android.graphics.Color;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
public class MainActivity extends AppCompatActivity {
private Button button2_;
private EditText editTextTextPersonName2_,editTextTextPersonName3_;
private ImageView imageView_;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button2_=findViewById(R.id. button2);
editTextTextPersonName2_=findViewById(R.id.editTextTextPersonName2);
imageView_=findViewById(R.id.imageView);
editTextTextPersonName3_=findViewById(R.id.editTextTextPersonName3);
}
Adding onclick function to the button and setting up the generated code to an ImageView
public void QRCodeButton(View view){
QRCodeWriter qrCodeWriter=new QRCodeWriter();
try {
BitMatrix bitMatrix=qrCodeWriter.encode((editTextTextPersonName2_.getText().toString() )+ (editTextTextPersonName3_.getText().toString()), BarcodeFormat.QR_CODE,200,200);
Bitmap bitmap= Bitmap.createBitmap(200,200,Bitmap.Config.RGB_565);
for(int x=0;x<200;x++){
for(int y=0;y<200;y++){
bitmap.setPixel(x,y,bitMatrix.get(x,y)? Color.BLACK:Color.WHITE);
}
}
imageView_.setImageBitmap(bitmap);
} catch (WriterException e) {
e.printStackTrace();
}
}
}
After running the program we are able to generate QR Code as shown in below figure.
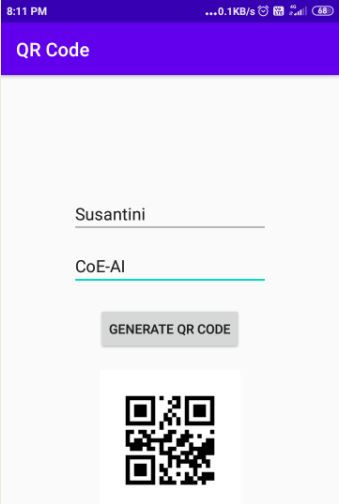
Figure 4:Android QR Code output
Source credits:Simplified Coding
Brought to you by-
CoE-AI(CET-BBSR)-An initiative by CET-BBSR,Tech Mahindra and BPUT to provide to solutions to REal world Problems through ML and IoT
Comments