Image Processing in android is a way that android developers use for quantitative data or numerical data sets to change the visual result. Image processing is done for various purposes, including the retrieval of specific information from an image, image recognition, image clarity or enhancement and pattern measurement.
When we talk about image processing in android we must be thinking how we can do it ?So bitmap is the java class which is used for the image processing in android.Bitmap is the simplest way to represent the image data.Bitmap divide the entire image into pixels....
Let an image is 16*16=256 pixels .Each pixel is either black or white .we can store the information using binary digits by representing the black pixel as 1 and white pixel as 0. 256/8=32bytes
Image resolution is a measure of the pixel density, given in dots per inch(dpi).Higher the resolution higher is the quality. The better the quality the memory requirement is more. These days most of the images are 24bits to describe the color of the images. Metadata(data about data) means the image files are slightly larger.
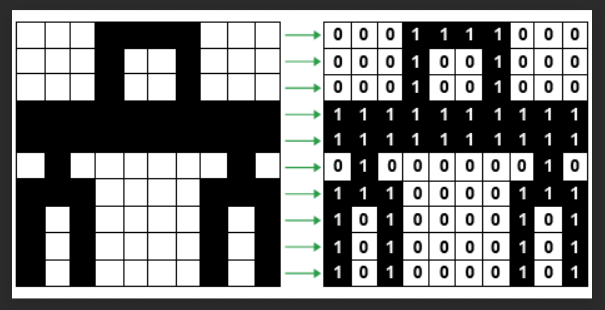
In this blog i have describe a workflow on text recognizer in Android using Google API-----
How image processing using Bitmap can be done in android is as follow-
After creating a new project in android studio in build.gradle (project)the google vision API need to be added by copying it from setup

This API dependency should be copy pasted in (build.gradle :app)
</activity>
<meta-data android:name="com.google.android.gms.vision.DEPENDENCIES"
android:value="orc"></meta-data>
</application>
In build gradle (OCR) the maven url is added
maven {
url "https://maven.google.com"
}
In manifest file the Internet permission and meta data must be added as below
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data android:name="com.google.android.gms.vision.DEPENDENCIES"
android:value="orc"></meta-data>
</application>
</manifest>
The designing of the android page where the image view will be given by the user to recognize the text in it using in just one click as below xml coding-
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ImageView
android:layout_height="300dp"
android:layout_width="250dp"
android:baselineAlignBottom="true"
android:layout_marginTop="90dp"
android:src="@drawable/b"
android:id="@+id/imageView"
/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:textAlignment="center"
android:gravity="center_horizontal"
android:layout_marginEnd="80dp"
android:layout_marginTop="23dp"
android:layout_below="@+id/imageView"
android:text="TextView"
android:layout_marginRight="88dp"
android:textSize="24sp"
android:id="@+id/textView2"/>
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:onClick="getTextFromImage"
android:layout_below="@+id/textView2"
android:layout_marginTop="19dp"
android:layout_alignParentLeft="true"
android:layout_alignParentBottom="true"
android:textAllCaps="false"
android:textSize="18sp"
android:text="View"
android:textColor="@android:color/white"
/>
</LinearLayout>
</ScrollView>
The java file will be designed in such a way that it will convert the input image into pixels..other wise it will give a toast message that "Could not get the text"
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.util.SparseArray;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.vision.Frame;
import com.google.android.gms.vision.text.TextBlock;
import com.google.android.gms.vision.text.TextRecognizer;
import org.w3c.dom.Text;
public class MainActivity extends AppCompatActivity {
ImageView i1;
TextView t1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
i1 = (ImageView)findViewById(R.id.imageView);
t1 = (TextView)findViewById(R.id.textView2);
}
public void getTextFromImage(View v)
{
Bitmap bitmap = BitmapFactory.decodeResource(getApplicationContext().getResources(),R.drawable.b);
TextRecognizer textRecognizer = new TextRecognizer.Builder(getApplicationContext()).build();
if (!textRecognizer.isOperational())
{
Toast.makeText(this, "Could not get the text", Toast.LENGTH_SHORT).show();
}
else
{
Frame frame = new Frame.Builder().setBitmap(bitmap).build();
SparseArray<TextBlock> items = textRecognizer.detect(frame);
StringBuilder sb = new StringBuilder();
for (int i=0; i<items.size();i++)
{
TextBlock myItem = items.valueAt(i);
sb.append(myItem.getValue());
sb.append("\n");
}
t1.setText(sb.toString());
}
}
}
The result of the app will be fetching all the text from the input image--
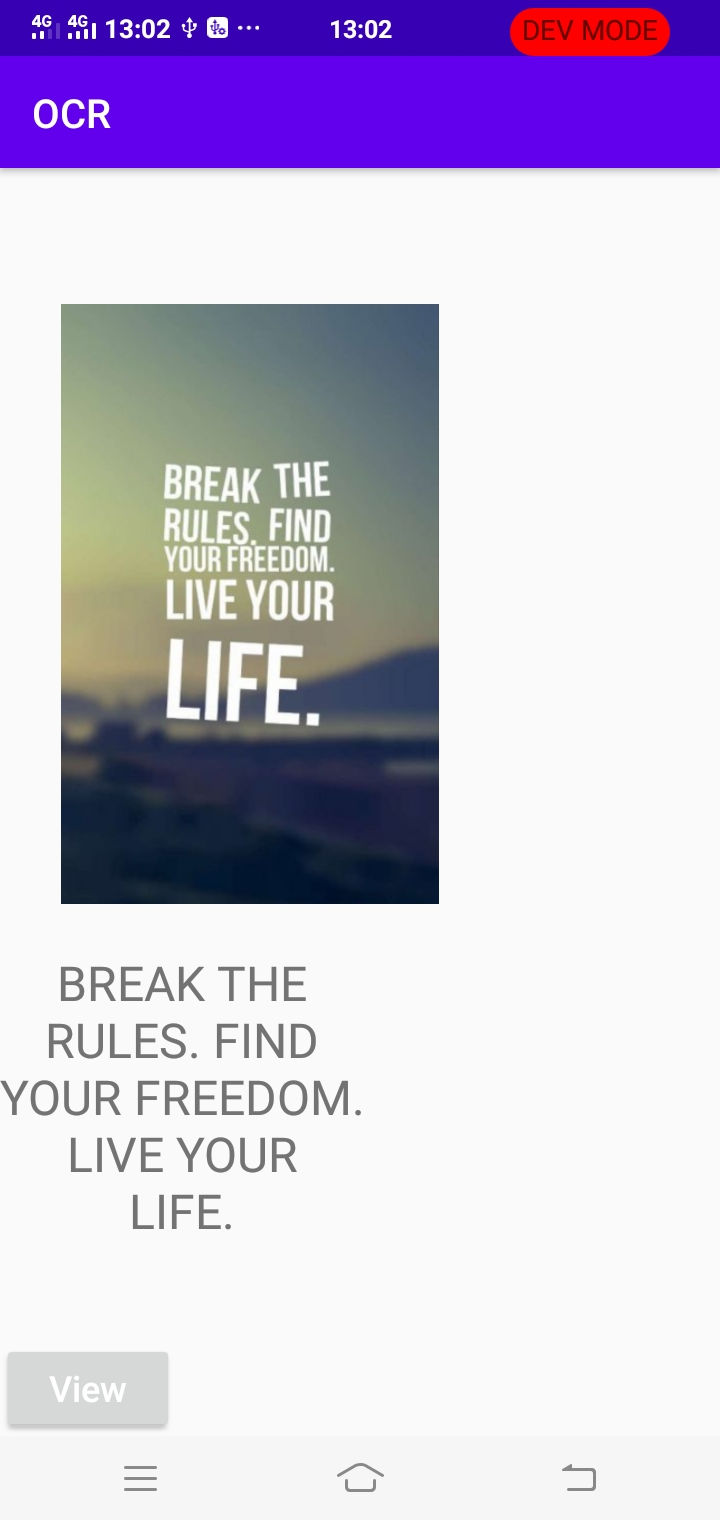
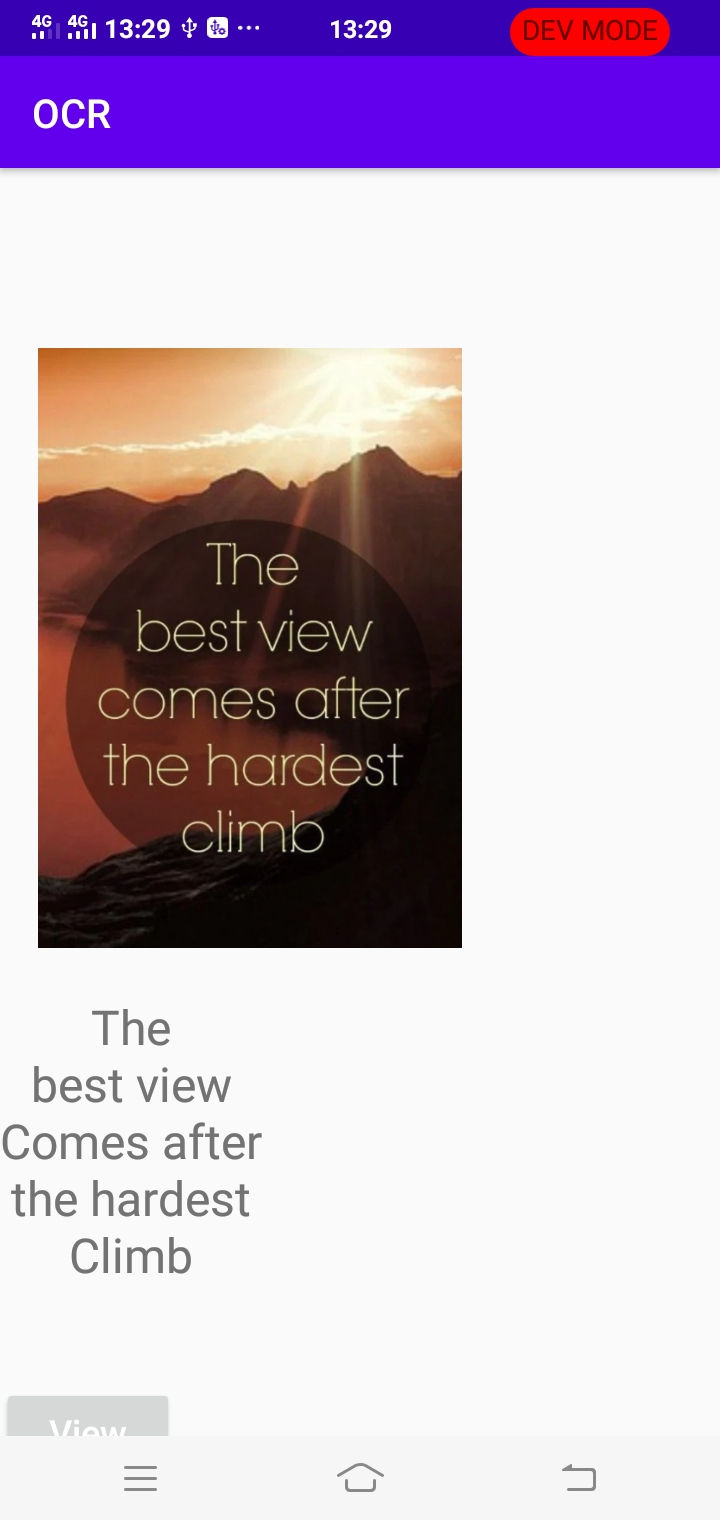
Uses of Bitmap in Android studio for Image Processing
-Image pixel manipulation
-Image Filtering
-Enhancement
-Blurring
-Character recognition
-Face ,fingerprint recognition
Brought to you By-
CoE-AI(CET-BBSR)-An initiative by CET-BBSR,Tech Mahindra and BPUT to provide to solutions to REal world Problems through ML and IoT
Comments